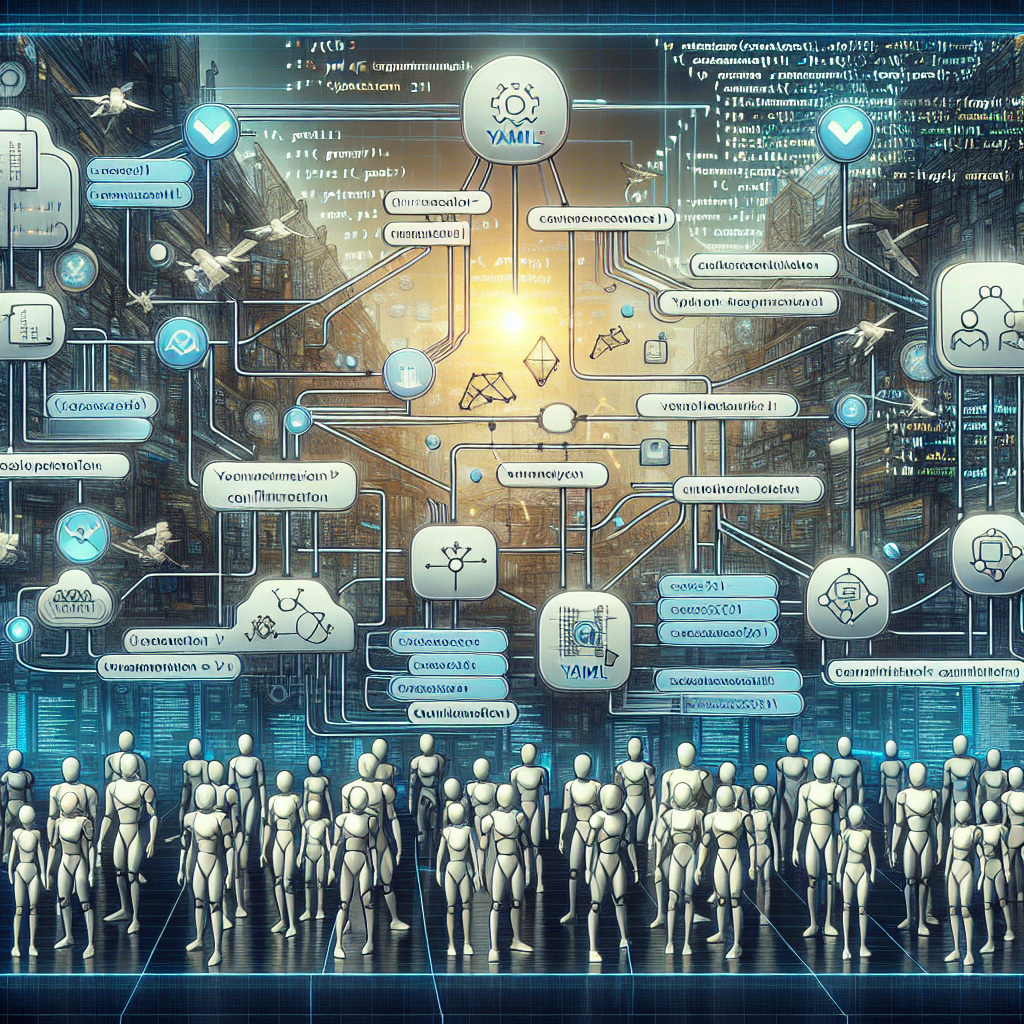
CrewAI introduces a unique and powerful mechanism for collaboration, known as “crews.” In this comprehensive guide, we will explore the concept of crews within CrewAI, the attributes that define them, how to create and execute them, and various features that enhance their functionality. This guide draws on detailed insights from the CrewAI documentation.
Definition of a Crew in CrewAI
A crew in CrewAI is essentially a collaborative group of “agents” that synergize to accomplish a set of tasks. Each crew not only defines a strategy for task execution but also manages agent collaboration and the overall workflow. This collaborative approach ensures efficient and organized task management, allowing for flexibility and scalability in operations.
Crew Attributes
Crews are defined by several key attributes:
- Tasks: The set of activities or assignments that the crew is responsible for executing.
- Agents: The actors or components within the crew that carry out the tasks.
- Process: This can be either sequential or hierarchical, dictating the order and dependencies of task execution.
- Verbose Logging: Enables detailed logging for debugging and auditing processes.
- Manager LLM: Utilized for hierarchical processes to oversee task execution.
- Language, Memory, Cache, and More: Optional configurations that enhance the functionality and efficiency of the crew by specifying language preferences, storing execution memories for decision-making, and caching results of executions.
Creating Crews
Crews can be created using YAML configuration files or directly through code. Utilizing YAML configuration offers a cleaner, more maintainable method that stays consistent with other project definitions within CrewAI, aligning with industry best practices.
Example of YAML Configuration
Here’s an example of how to structure crews using code:
from crewai import Agent, Crew, Task, Process
from crewai.project import CrewBase, agent, task, crew, before_kickoff, after_kickoff
@CrewBase
class YourCrewName:
agents_config = 'config/agents.yaml'
tasks_config = 'config/tasks.yaml'
@before_kickoff
def prepare_inputs(self, inputs):
inputs['additional_data'] = "Some extra information"
return inputs
@after_kickoff
def process_output(self, output):
output.raw += "\nProcessed after kickoff."
return output
@agent
def agent_one(self) -> Agent:
return Agent(config=self.agents_config['agent_one'], verbose=True)
@task
def task_one(self) -> Task:
return Task(config=self.tasks_config['task_one'])
@crew
def crew(self) -> Crew:
return Crew(agents=self.agents, tasks=self.tasks, process=Process.sequential, verbose=True)
This snippet demonstrates a YAML-based approach, ensuring clarity and ease of maintenance across your CrewAI projects.
Crew Output
Once a crew completes its tasks, the output is encapsulated within the CrewOutput
class. This class provides structured results in various formats, including raw strings, JSON, and Pydantic models. Additionally, it provides insights into token usage, helping analyze agent performance.
Memory and Cache Utilization
To enhance operational efficiency, crews can utilize memory and cache:
- Memory: Stores execution memories, aiding in more informed decision-making by referencing past experiences.
- Cache: Stores results from executed tools, reducing redundant computing by reusing previous outputs.
Execution Processes
CrewAI supports two primary execution processes:
- Sequential: Tasks are executed one after the other, ensuring linear progression.
- Hierarchical: Managed by a “manager agent,” this process requires validation steps and functionalities for tasks, often used for complex task management scenarios.
Kicking Off a Crew
To initiate task execution, CrewAI provides several methods:
- Using
kickoff()
, which starts the immediate execution of tasks. - Alternatives like
kickoff_for_each()
,kickoff_async()
, andkickoff_for_each_async()
offer varied styles, enhancing flexibility in task management and execution.
Replaying Tasks
CrewAI’s CLI includes a replay
command that allows for the replay of tasks from specific points. This feature preserves the previous execution context and is facilitated through commands like crewai log-tasks-outputs
and crewai replay -t <task_id>
, ensuring continuity and integrity.
Decorators and Code Organization
The CrewAI framework leverages several decorators, including @CrewBase
, @agent
, @task
, and @crew
. These decorators simplify project management by automatically collecting agents and tasks, promoting organized and maintainable code structures.
In conclusion, understanding and utilizing crews within CrewAI can significantly enhance collaborative task management and execution. By leveraging the attributes and features discussed here, users can effectively deploy, monitor, and optimize their workflows in a variety of contexts. This guide, based on CrewAI documentation, offers all the essential insights needed to master crews in CrewAI.