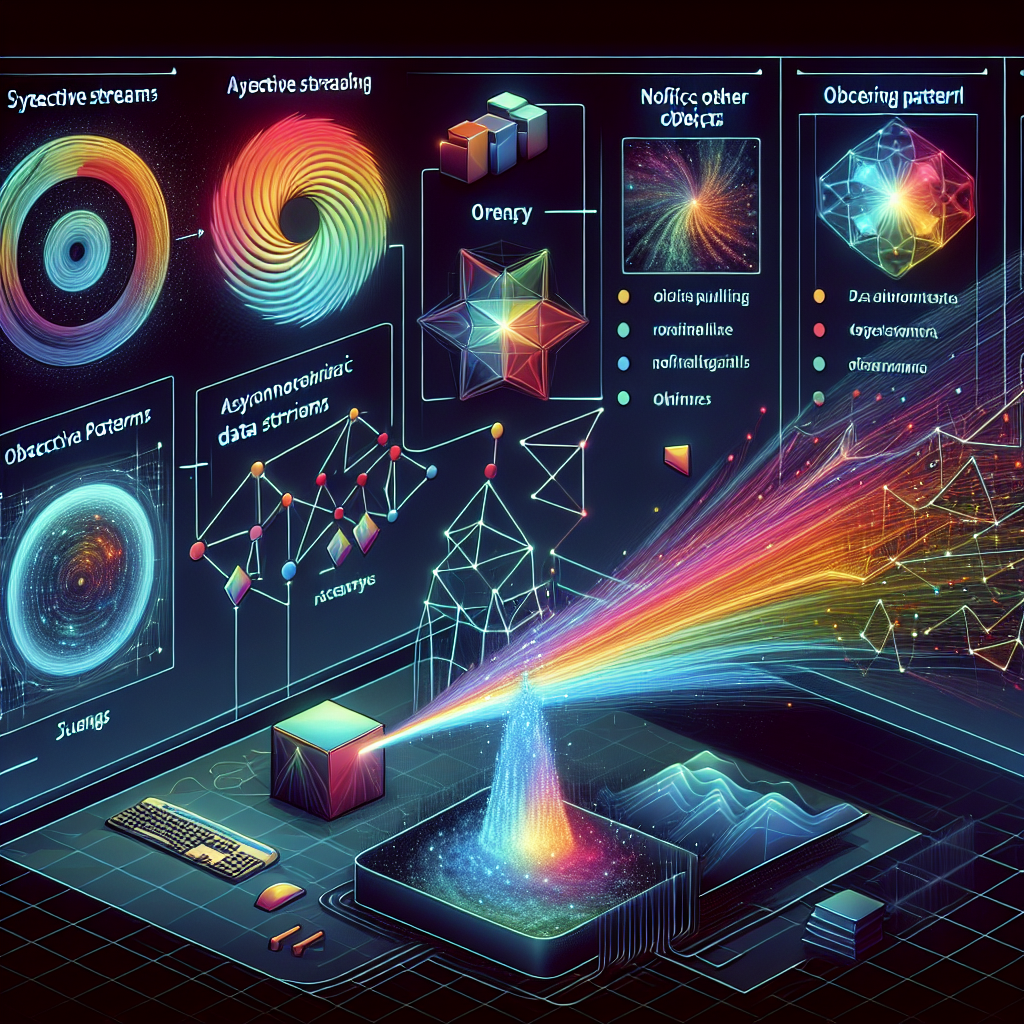
What is Reactive Programming?
Reactive Programming is a programming paradigm centered on asynchronous data streams and the propagation of change.
It allows developers to create systems that are highly responsive and resilient, making them particularly well-suited to dynamic environments where non-blocking mechanisms are crucial for performance.
Example: Asynchronous Event Handling in Go
package main
import (
"fmt"
"time"
)
func main() {
events := make(chan string)
go func() {
time.Sleep(1 * time.Second)
events <- "Event Triggered"
}()
fmt.Println("Waiting for event...")
fmt.Println(<-events)
}
This code snippet demonstrates how events are handled asynchronously, allowing the program to continue running
while waiting for the event to trigger.
RxGo: Reactive Extensions for Go
RxGo brings the powerful paradigm of Reactive Extensions (Rx) to Go. It provides an abstraction for working with asynchronous and event-driven programs by using concepts such as observables and operators.
Example: Basic RxGo Usage
package main
import (
"fmt"
"github.com/reactivex/rxgo/v2"
)
func main() {
observable := rxgo.Just(1, 2, 3)().Observe()
for item := range observable {
fmt.Println(item.V)
}
}
Implementing the Observer Pattern
The Observer pattern is pivotal to Reactive Programming, establishing a subscription mechanism to notify multiple observers about changes to an object.
Example: Observer Pattern Implementation
package main
import "fmt"
type Observer interface {
Update(string)
}
type ConcreteObserver struct {
id int
}
func (co *ConcreteObserver) Update(message string) {
fmt.Printf("Observer %d received message: %s\n", co.id, message)
}
type Subject struct {
observers []Observer
}
func (s *Subject) AddObserver(o Observer) {
s.observers = append(s.observers, o)
}
func (s *Subject) NotifyAll(message string) {
for _, observer := range s.observers {
observer.Update(message)
}
}
func main() {
obs1 := &ConcreteObserver{id: 1}
obs2 := &ConcreteObserver{id: 2}
subject := &Subject{}
subject.AddObserver(obs1)
subject.AddObserver(obs2)
subject.NotifyAll("Event 1")
}
This pattern ensures that the system reacts to changes in state effectively, notifying all dependent components.
Using Channels for Reactive Streams
package main
import "fmt"
func main() {
stream := make(chan int)
go func() {
for i := 0; i < 5; i++ {
stream <- i
}
close(stream)
}()
for value := range stream {
fmt.Println(value)
}
}
This example shows how values are processed in a non-blocking manner.
Reactive Frameworks in Go
Go offers various frameworks to build non-blocking, event-driven applications. Two noteworthy examples include:
- Fyne: A GUI framework to build lightweight interfaces swiftly.
- Gorilla Websocket: A toolkit for dealing with websockets in a scalable manner.
Example: Integrating Gorilla Websocket
package main
import (
"github.com/gorilla/websocket"
"net/http"
"log"
)
var upgrader = websocket.Upgrader{}
func handleConnection(w http.ResponseWriter, r *http.Request) {
conn, err := upgrader.Upgrade(w, r, nil)
if err != nil {
log.Println("Error upgrading connection:", err)
return
}
defer conn.Close()
for {
messageType, message, err := conn.ReadMessage()
if err != nil {
log.Println("Error reading message:", err)
break
}
log.Printf("Received message: %s", message)
if err := conn.WriteMessage(messageType, message); err != nil {
log.Println("Error writing message:", err)
break
}
}
}
func main() {
http.HandleFunc("/ws", handleConnection)
log.Fatal(http.ListenAndServe(":8080", nil))
}
Error Handling in Reactive Programming
Handling errors gracefully is critical in Reactive Programming as it ensures system robustness and reliability.
Example: Error Handling with RxGo
package main
import (
"fmt"
"github.com/reactivex/rxgo/v2"
"errors"
)
func main() {
items := []interface{}{1, 2, 3, errors.New("error 4")}
observable := rxgo.From(items).Catch(func(err error) {
fmt.Println("Error caught:", err)
}).Observe()
for item := range observable {
if item.E != nil {
fmt.Println("Received an error:", item.E)
} else {
fmt.Println("Next item:", item.V)
}
}
}
Chaining Events
One of the strengths of reactive programming is the ability to chain operations on data streams, allowing complex data transformations in a concise manner.
Example: Chaining with RxGo
package main
import (
"fmt"
"github.com/reactivex/rxgo/v2"
)
func main() {
obs := rxgo.From([]int{1, 2, 3, 4}).
Map(func(item interface{}) interface{} {
return item.(int) * 2
}).Observe()
for item := range obs {
fmt.Println("Doubled value:", item.V)
}
}
Combining Streams
Reactive Programming provides mechanisms to merge multiple streams, enabling concurrent data processing.
Example: Merging Observables with RxGo
package main
import (
"fmt"
"github.com/reactivex/rxgo/v2"
)
func main() {
obs1 := rxgo.From([]interface{}{1, 2, 3})
obs2 := rxgo.From([]interface{}{4, 5, 6})
merged := rxgo.Merge(obs1, obs2).Observe()
for item := range merged {
fmt.Println("Merged item:", item.V)
}
}
Using Backpressure Strategies
In any reactive system, handling backpressure — where the production of data outpaces consumption — is crucial for system health and stability.
Example: Backpressure with Buffered Channels
package main
import (
"fmt"
"time"
)
func producer(stream chan<- int) {
for i := 0; i < 10; i++ {
fmt.Println("Producing:", i)
stream <- i
}
close(stream)
}
func consumer(stream <-chan int) {
for item := range stream {
fmt.Println("Consuming:", item)
time.Sleep(1 * time.Second) // Simulating slow consumer
}
}
func main() {
stream := make(chan int, 3) // Buffered channel with a capacity of 3
go producer(stream)
consumer(stream)
}
Testing Reactive Systems
Testing in a reactive context requires careful design to handle asynchronous behaviors reliably.
- Testify: A Go library that facilitates writing expressive and informative tests, especially
useful for reactive systems.
Example: Testing Asynchronous Code
package main
import (
"testing"
"github.com/stretchr/testify/assert"
)
func TestAsyncFunction(t *testing.T) {
stream := make(chan int, 1)
go func() {
stream <- 10
close(stream)
}()
value := <-stream
assert.Equal(t, 10, value, "They should be equal")
}
In conclusion, Reactive Programming with Go opens up a world of possibilities for creating efficient, responsive applications. By leveraging Go’s concurrency primitives and various reactive extensions and frameworks, developers can build systems that respond to events seamlessly while maintaining robustness and scalability.