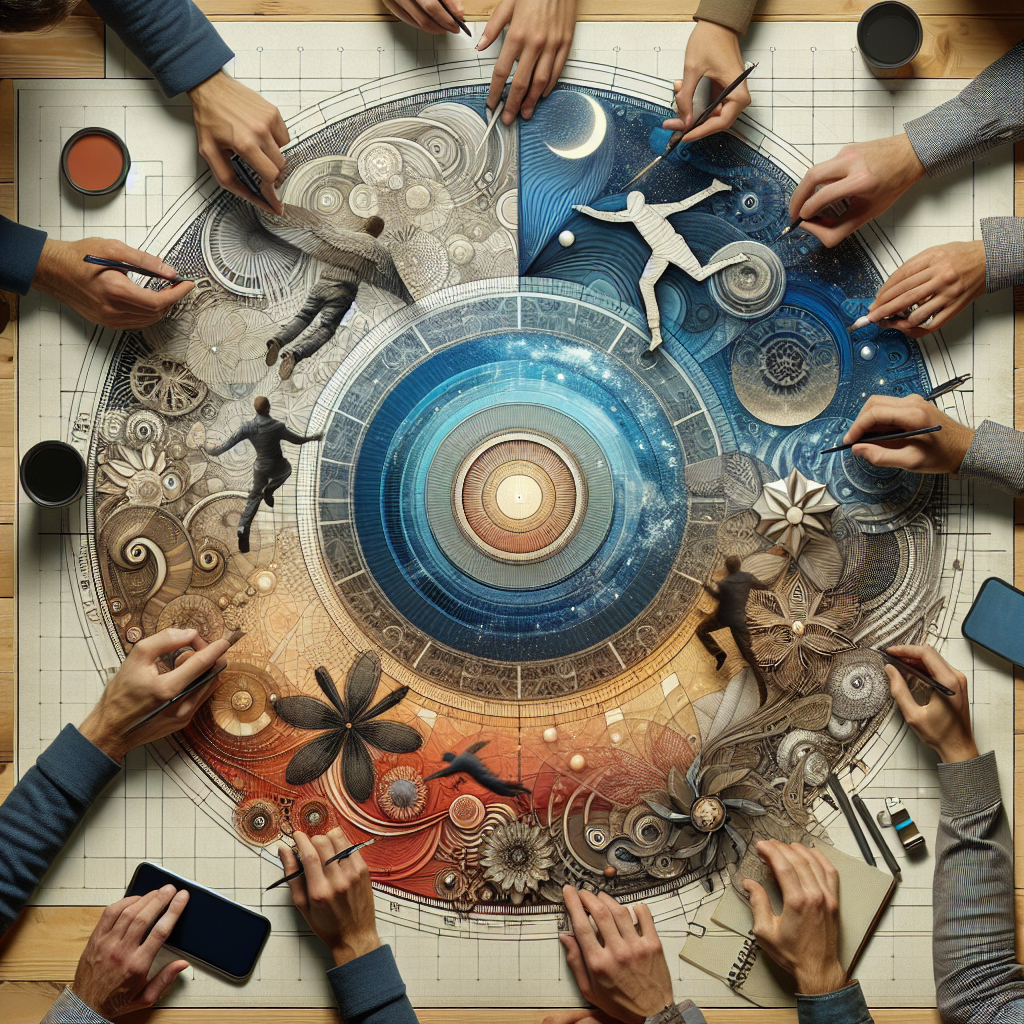
Image Credit: This image was created by DALL·E AI.
Understanding and Creating Crews in CrewAI
As organizations increasingly harness the power of AI, effective team management among AI agents becomes essential. CrewAI offers an excellent framework for managing these AI agents—referred to as Crews—to optimize task completion. This guide walks you through key aspects of setting up and using Crews in CrewAI.
What is a Crew?
A Crew in CrewAI is a collaborative group of AI agents working together to complete tasks. Each agent is assigned a specific role that contributes to achieving the collective goal efficiently, similar to a human team where each member adds unique expertise.
Key Crew Attributes
- Tasks: Specific jobs or responsibilities assigned to the Crew.
- Agents: The collective of AI agents forming the Crew.
- Process: Describes how tasks are executed, e.g., sequential or hierarchical.
- Verbose: Controls the level of detail in execution logs.
- Manager LLM: Specifies the language model for managing agents in a hierarchical structure.
Using YAML for Configuration
The first step in deploying a Crew involves defining agents and tasks using YAML files, which provide clarity and structure.
Agents Configuration
agent_one:\n role: "Data Analyst"\n goal: "Analyze market data."
Tasks Configuration
task_one:\n description: "Collect recent market data."\n expected_output: "A report summarizing key trends."
Building the Crew Class
Using decorators, Crew classes efficiently organize agents and tasks for execution.
from crewai import Agent, Crew, Task\n\n@CrewBase\nclass MyCrew:\n @agent\n def agent_one(self) -> Agent:\n return Agent(name='agent_one', role='Data Analyst', goal='Analyze market data.')\n\n @task\n def task_one(self) -> Task:\n return Task(name='task_one', description='Collect recent market data.')
Executing the Crew
After setting up your Crew, execution can begin with the kickoff()
method.
result = my_crew.kickoff()\nprint(result)
Accessing and Analyzing Outputs
Upon completion of tasks, retrieving results effectively is crucial.
crew_output = my_crew.kickoff()\nprint(f"Raw Output: {crew_output.raw}")
Ensuring Secure Execution
Security is vital when it comes to agent code execution, allowing only verified actions:
secure_agent = Agent(\n role="System Administrator",\n allow_code_execution=True,\n code_execution_mode="secure"\n)
Organizing Hierarchical Task Delegation
For organized task management, a hierarchical framework can be established with a managing agent:
from crewai import Process, ManagerAgent\n\n@crew\ndef crew(self) -> Crew:\n return Crew(\n agents=[self.agent_one(), self.agent_two()],\n process=Process.hierarchical,\n manager_agent=ManagerAgent(),\n )
Conclusion
CrewAI provides an efficient framework for managing AI agent teams, enhancing productivity and success in task completion. Leveraging YAML for setup, decorators for organization, and built-in security, CrewAI is a robust solution for AI task management. Explore the CrewAI documentation for more insights and advanced configurations.